Python is one of the most used programming languages in the world, and that can be contributed to its general-purpose nature, which makes it a suitable candidate for various domains in the industry. With Python, you can develop programs not just for the web, but also for desktop and command-line. Python can be suitable for programmers of varying skill levels, right from the students to intermediate developers, to experts and professionals. But every programming language requires constant learning, and its the same case with Python especially if you’re going to use it create websites. But if you don’t have the time to learn it to setup your site, you can visit this website to know more about our services.
If you truly want to get in-depth practical knowledge, there is no better way to get your hands dirty with Python than to undertake some cool projects that will not only keep you occupied in your free time but will also teach you how to get more out of Python.
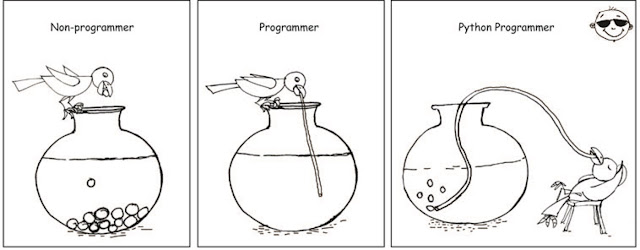
Table of Contents
10 Easy and Fun Projects to kick start your Python Learning
So, in this article, we will discuss Ten interesting Python projects for beginners. It sounds cool. Isn’t it?
Basic Requirement
- You must have Python 3 installed.
- You must be familiar with basic Python and executing Python programs.
- You can use IDLE or any Python IDE to run these programs.
- You have to use Command Prompt to install modules using pip. Example : pip install instapy
Rest, you can simply copy paste the code and see the Python magic !
Download Profile Picture of any Instagram Account
So lets say you have to download profile pictures of your favorite celebs, Sachin Tendulkar in this case, then this 4 line program will automatically do that for you.
First install via Command Prompt : pip install instaloader. Then run the below script in any IDE, or Python default IDLE.
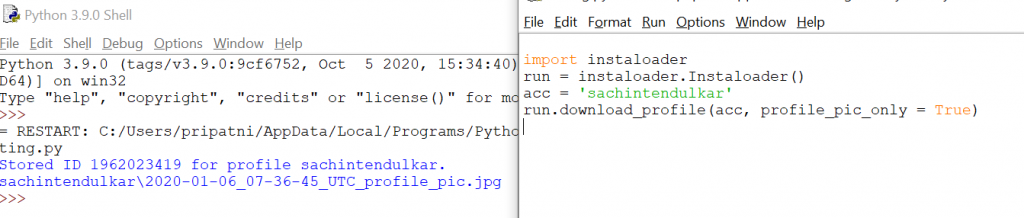
Here is the code you can copy :
import instaloader
run = instaloader.Instaloader()
acc = 'sachintendulkar'
run.download_profile(acc, profile_pic_only = True)
Output
Stored ID 1962023419 for profile sachintendulkar.
sachintendulkar\2020-01-06_07-36-45_UTC_profile_pic.jpg
In your default Python folder, you will find a new folder with the name ‘sachintendulkar’ and will have the Profile image of Sachin along with a few files that you can ignore.
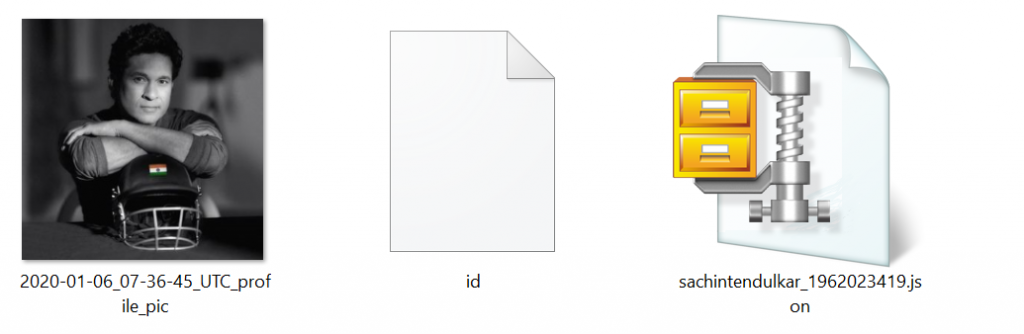
Send Automated Email from your Gmail ID
Yes, you can automate your mails via Python. It feels like first level of hacking, right? Dont worry it is totally legal !
It is important to turn Less Secure Apps Access ‘ON’ before executing the script. Do it from here.
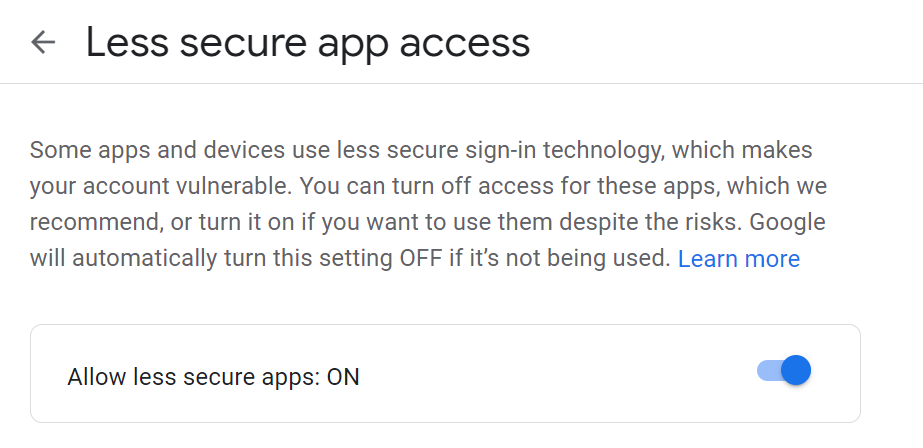
Check stackoverflow and other google help if you face issues, but this method works 100%. Now copy the below code, enter your mail details (mail id and password), and check your inbox for the magic !
import smtplib
s = smtplib.SMTP('smtp.gmail.com', 587) # creates SMTP session
s.starttls() # start TLS for security
s.login("<Email ID>", "<Password>")
message = """\
Subject: Hi there
This message is sent from Python."""
s.sendmail("<From Email ID", "<To Email ID>", message)
s.quit()
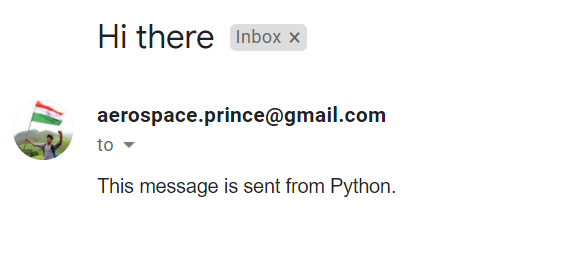
Download Images for a particular Instagram hashtag
Lets say you need a few images on a particular hashtag from Instagram. Its as easy as 3 lines of code !
import instaloader
loader = instaloader.Instaloader(download_videos=False, save_metadata=False, post_metadata_txt_pattern='')
loader.download_hashtag('python', max_count=10)
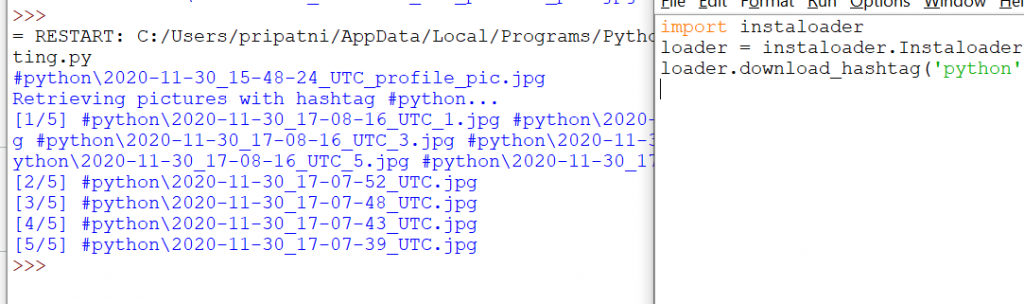
This code will create a new folder with name #python and will have 10 latest images with #python.

Want to learn Data Science – the best career option in 20th Century ? Read all about it !
Draw a Heart and fill with Red Color
Its a simple cute exercise that will animate a heart shape and fill with red color. Yes, its cute !
import turtle
turtle.speed(3)
turtle.pensize(3)
def func():
for i in range(200):
turtle.right(1)
turtle.forward(1)
turtle.color('black', 'red')
turtle.begin_fill()
turtle.left(140)
turtle.forward(111.65)
func()
turtle.left(120)
func()
turtle.forward(111.65)
turtle.end_fill()
turtle.hideturtle()
turtle.done()
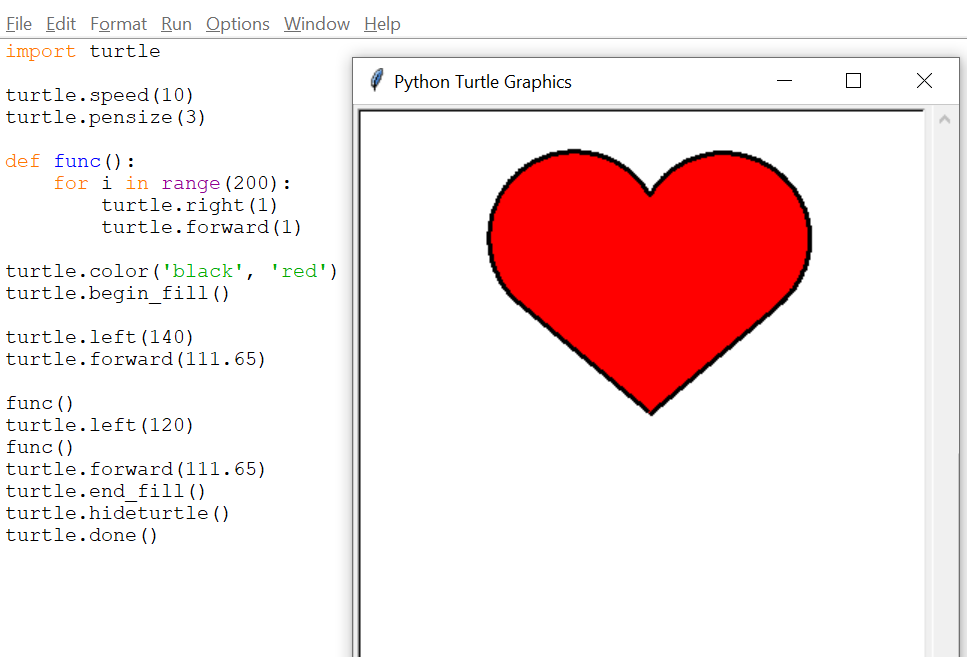
Create Random one-liner Programming jokes
You might want to generate random jokes for your website or portfolio. This module pyjokes lets you do that comfortably.
pip install pyjokes
import pyjokes
jokes = pyjokes.get_jokes(language='en', category= 'neutral')
for i in range(5):
print(i+1,".",jokes[i])
Here is the Output :
= RESTART: C:/Users/pripatni/AppData/Local/Programs/Python/Python39/Practice/testing.py
1 . Complaining about the lack of smoking shelters, the nicotine addicted Python programmers said there ought to be 'spaces for tabs'.
2 . Ubuntu users are apt to get this joke.
3 . Obfuscated Reality Mappers (ORMs) can be useful database tools.
4 . Asked to explain Unicode during an interview, Geoff went into detail about his final year university project. He was not hired.
5 . Triumphantly, Beth removed Python 2.7 from her server in 2030. 'Finally!' she said with glee, only to see the announcement for Python 4.4.
>>>
Post a Photo to your Instagram profile
Yes, you can automate posting pictures to your Instagram account. Instabot lets you do that quickly and works 100% !
From command line run pip install instabot and then copy the below code in your IDE. The image to upload should be in your python working directory, or you have to specify the full path. Enter your Instagram credentials where asked.
from instabot import Bot
bot = Bot()
bot.login(username = "<Enter your username>",
password = "<Enter your Password>")
bot.upload_photo("udemy_certificate.jpg",
caption = "Test")
Run the code and check your Instagram !
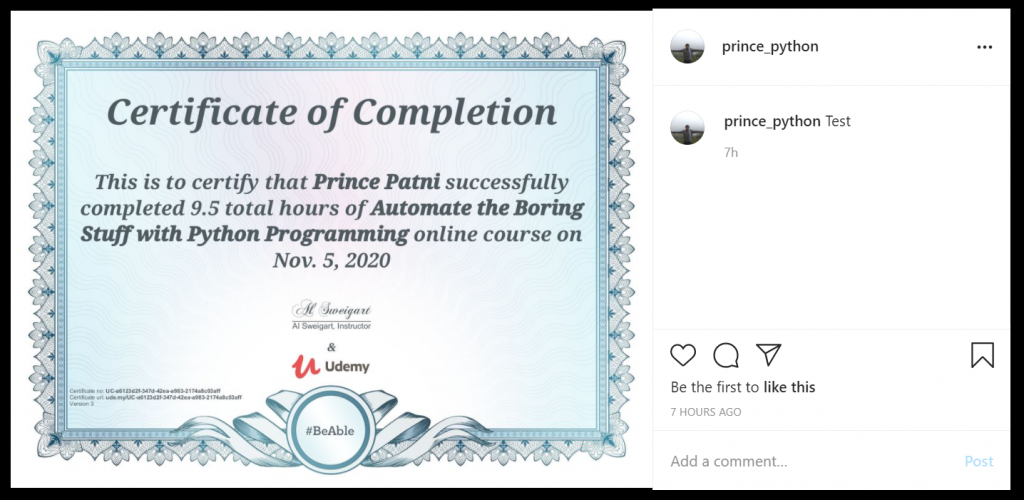
Check out these best Web Development Courses for your next career jump !
Create your Website’s or Social Media Profile’s QR Code
import pyqrcode
data = 'https://www.instagram.com/eat.party.repeat/'
img = pyqrcode.create(data)
img.png('myqrcode.png', scale= 10)
Output will be a png image of your link’s QR code. This code can be scanned by Google Lens or any other QR Code scanner app and will redirect the user to this link once clicked.
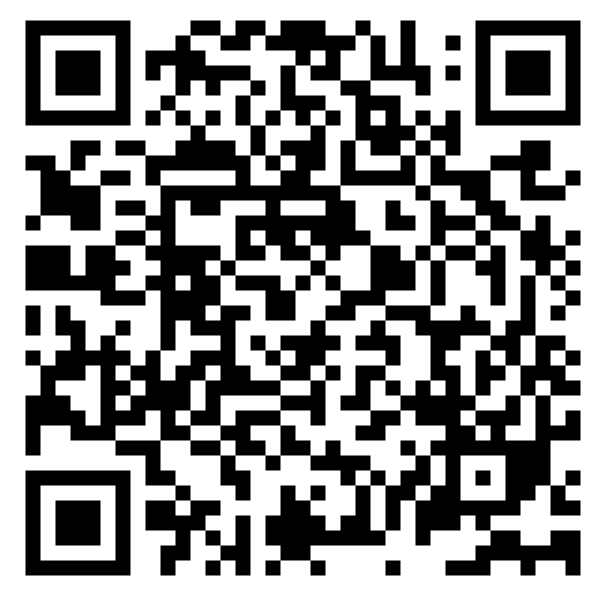
Create Captcha of your Name
Captchas are irritating but are important from security perspective. From command line, run pip install captcha and then paste the below code in your IDE. It will create a captcha of the word that you give and save in an image format in the root folder.
from captcha.image import ImageCaptcha
image = ImageCaptcha(width = 280, height = 90)
data = image.generate('princepatni')
image.write('princepatni', 'prince_captcha.png')
This is what I got for my name. Try yours !
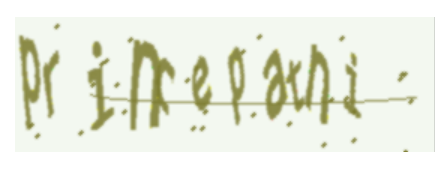
Make a Digital Clock
Use his code to create a digital clock that runs on your desktop, giving you the pleasure of seeing something now and then that you have made !
from tkinter import *
from tkinter.ttk import *
from time import strftime
root = Tk()
root.title('Digital clock')
def clock():
tick = strftime('%H:%M:%S %p')
label.config(text =tick)
label.after(1000, clock)
label = Label(root, font =('sans', 80), background = 'red', foreground = 'black')
label.pack(anchor= 'center')
clock()
mainloop()
This is what it looks like. Play with its background and foreground colors to suit your mood.
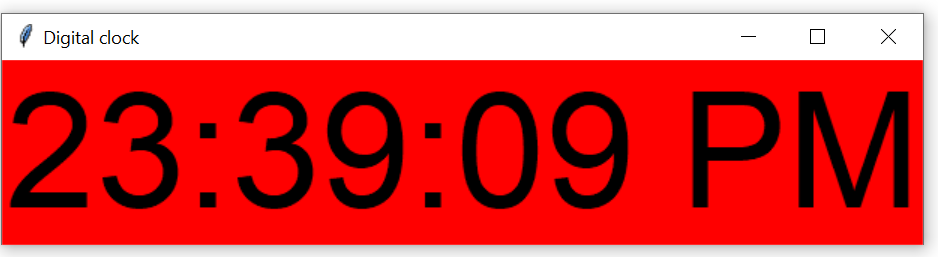
A Complete Dashboard Development Guide that you must have handy !
Create a Twitter Bot
How about writing a script that logs in a twitter account, look for a particular hashtag, and automatically follows top accounts on that word. Not just that, it will retweet top tweets, favorite them, and even reply to them !
This can be so good for your social media marketing campaigns, without having to do much, just run a script !
Use the command prompt to install tweepy and tkinter modules. Then go to Twitter – https://developer.twitter.com/en/apps – If you dont have a developer account you have to apply for one and wait for a day or two. (Dont worry, you dont have to be a twitter developer to get a developer account on twitter, anyone can apply and get one.) From the above page, go to Create An App – Keys & Tokens. There you will find these 4 tokens that are important for this python script to run.
Once you have these, running the below script is a breeze and you don’t have to input anything else. Once you execute the script it will open a GUI where you have to input the target keyword, and answer a few simple questions – and thats it ! Check your twitter account to see that magic is done !
import tweepy
from tkinter import *
consumer_key = '<Enter here>'
consumer_secret = '<Enter here>'
access_token = '<Enter here>'
access_token_secret = '<Enter here>'
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
user = api.me()
print (user.name)
print(user.location)
for follower in tweepy.Cursor(api.followers).items():
follower.follow()
print("Followed everyone that is following " + user.name)
root = Tk()
label1 = Label( root, text="Search")
E1 = Entry(root, bd =5)
label2 = Label( root, text="Number of Tweets")
E2 = Entry(root, bd =5)
label3 = Label( root, text="Response")
E3 = Entry(root, bd =5)
label4 = Label( root, text="Reply?")
E4 = Entry(root, bd =5)
label5 = Label( root, text="Retweet?")
E5 = Entry(root, bd =5)
label6 = Label( root, text="Favorite?")
E6 = Entry(root, bd =5)
label7 = Label( root, text="Follow?")
E7 = Entry(root, bd =5)
def getE1():
return E1.get()
def getE2():
return E2.get()
def getE3():
return E3.get()
def getE4():
return E4.get()
def getE5():
return E5.get()
def getE6():
return E6.get()
def getE7():
return E7.get()
def mainFunction():
getE1()
search = getE1()
getE2()
numberOfTweets = getE2()
numberOfTweets = int(numberOfTweets)
getE3()
phrase = getE3()
getE4()
reply = getE4()
getE5()
retweet = getE5()
getE6()
favorite = getE6()
getE7()
follow = getE7()
if reply == "yes":
for tweet in tweepy.Cursor(api.search, search).items(numberOfTweets):
try:
#Reply
print('\nTweet by: @' + tweet.user.screen_name)
print('ID: @' + str(tweet.user.id))
tweetId = tweet.user.id
username = tweet.user.screen_name
api.update_status("@" + username + " " + phrase, in_reply_to_status_id = tweetId)
print ("Replied with " + phrase)
except tweepy.TweepError as e:
print(e.reason)
except StopIteration:
break
if retweet == "yes":
for tweet in tweepy.Cursor(api.search, search).items(numberOfTweets):
try:
#Retweet
tweet.retweet()
print('Retweeted the tweet')
except tweepy.TweepError as e:
print(e.reason)
except StopIteration:
break
if favorite == "yes":
for tweet in tweepy.Cursor(api.search, search).items(numberOfTweets):
try:
#Favorite
tweet.favorite()
print('Favorited the tweet')
except tweepy.TweepError as e:
print(e.reason)
except StopIteration:
break
if follow == "yes":
for tweet in tweepy.Cursor(api.search, search).items(numberOfTweets):
try:
#Follow
tweet.user.follow()
print('Followed the user')
except tweepy.TweepError as e:
print(e.reason)
except StopIteration:
break
submit = Button(root, text ="Submit", command = mainFunction)
label1.pack()
E1.pack()
label2.pack()
E2.pack()
label3.pack()
E3.pack()
label4.pack()
E4.pack()
label5.pack()
E5.pack()
label6.pack()
E6.pack()
label7.pack()
E7.pack()
submit.pack(side =BOTTOM)
root.mainloop()
The GUI looks something like this, its a simple one but can be made into a beautiful interface.
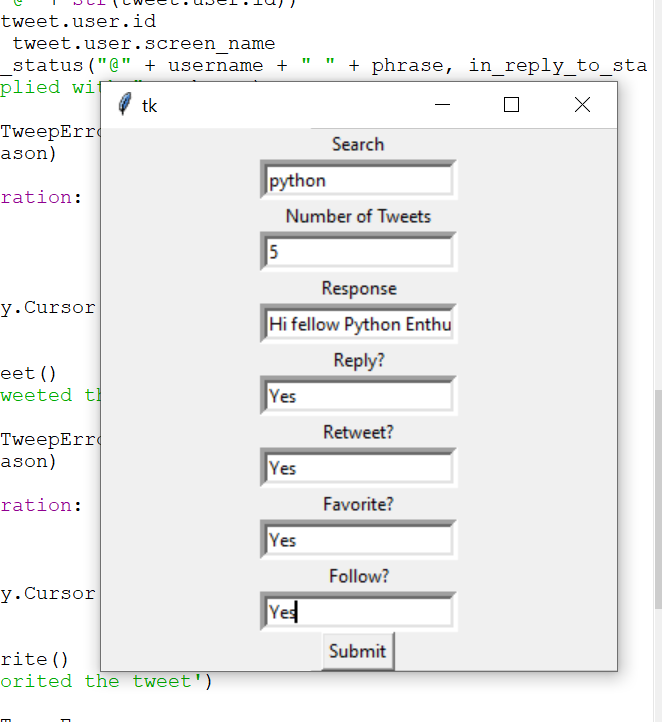
Enter values in input boxes like above and click Submit. Check your twitter profile and see the Tweets, Tweets with Replies, Likes etc to see what script did effortlessly !
Hope you enjoyed doing these small, yet fun exercises and feel the immense power python has !
The above Ten python projects are helpful to introduce practical application through the Python programming language. Implementing these projects introduces important concepts such as file handling and GUI design to a beginner programmer. You can simply open IDLE, copy the code and see the magic !
Concluding our list of a handful of interesting ideas and projects you can build using Python, we can say that Python can be a very useful programming language to develop applications of all sorts and scales. To wrap things up, I would like to say the potential with Python is limitless, and the only thing that you might be missing could be the right idea !
Lets just look at Python a little more !
Python Applications
Some of the applications of Python programming language are as follows:
- Web development: Python is widely used for developing web development. Python provides a number of frameworks that make it easier for developers to build these projects.
- Game development: Python provides a lot flexibility for developers to develop game projects. Python provides number of libraries such as PySoy and PyGame can be used widely for game development.
- Machine Learning and Artificial Intelligence: Machine Learning and Artificial Intelligence projects are different from traditional software projects. To develop such projects, it requires a programming language that is more stable, secure, platform independent, flexible and it must be equipped with tools that can handle various unique requirements. Python programming language has such features that makes it most suited programming language to develop ML and AI projects.
- Desktop GUI: Python offers many GUI toolkits and frameworks which makes it the most preferred programming language to develop desktop applications. Some of the libraries such as PyQt, PyGtk, Tkinter allow developers to create highly functional Graphical User Interfaces.
- Audio and video applications: Python offers such features that developers can use this programming language to develop Audio and Video applications. Some of the applications such as TimPlayer and Cplay have been developed using Python.
- Business applications: Python can be used to develop different business applications covering different domains such as e-commerce, ERP etc. Since developing such applications require a programming language which is scalable, extensible, readable etc. and Python offers such applications.
Starting your own Website is as easy as buying a coffee. Know how !
Python Advantages
- Easy to learn: Since Python follows very simple coding structure, few keywords and has a clearly defined syntax, it is considered as one of the easiest programming to learn.
- Object-Oriented language: Python supports Object-Oriented programming concept. It supports Inheritance, Polymorphism, Encapsulationetc. This feature helps programmers to write reusable code and develop applications in lesser code.
- Provides large standard library: Python provides large number of libraries that has some handy codes nd functions making it easy for programmers to build projects.
- Cross-platform language: Python is cross-platform programming language. This programming language can run on different platforms such as Windows, Linux, Unix and Macintosh etc. This helps programmers to run develop the software for several competing platforms by writing a program only one time.
- Interpreted: Python is an interpreted programming language. This means Python program is executed one line at a time. This makes debugging easy and portable.
- High-Level Language: Python has been designed to be a high-level programming language. It means while writing Python codes developers don’t need to be aware of the coding structure, architecture as well as memory management.
Best Python Courses
Learn Python Programming Masterclass
- Have a fundamental understanding of the Python programming language.
- Have the skills and understanding of Python to confidently apply for Python programming jobs.
- Acquire the pre-requisite Python skills to move into specific branches – Machine Learning, Data Science, etc..
- Add the Python Object-Oriented Programming (OOP) skills to your résumé.
- Understand how to create your own Python programs.
- Learn Python from experienced professional software developers.
- Understand both Python 2 and Python 3.
The Python Mega Course: Build 10 Real World Applications
- Go from a total beginner to a confident Python programmer
- Create 10 real-world Python programs (no toy programs)
- Strengthen your skills with bonus practice activities throughout the course
- Create an English Thesaurus app that returns definitions of English words
- Create a personal website entirely in Python
- Create a mobile app that improves your mood
- Create a portfolio website and publish it on a real server
- Create a desktop app for storing data for books
- Create a webcam app that detects moving objects
- Create a web scraper that extracts real-estate data
- Create a data visualization app
- Create a database app
- Create a geocoding web app
- Send automated emails
- Analyze and visualize data
- Use Python to schedule programs based on computer events.
- Learn OOP (Object-Oriented Programming)
- Learn GUIs (Graphical-User Interfaces)
2020 Complete Python Bootcamp From Zero to Hero in Python
- Learn to use Python professionally, learning both Python 2 and Python 3!
- Create games with Python, like Tic Tac Toe and Blackjack!
- Learn advanced Python features, like the collections module and how to work with timestamps!
- Learn to use Object Oriented Programming with classes!
- Understand complex topics, like decorators.
- Understand how to use both the Jupyter Notebook and create .py files
- Get an understanding of how to create GUIs in the Jupyter Notebook system!
- Build a complete understanding of Python from the ground up!
The Python Bible™ | Everything You Need to Program in Python
- Gain a Solid & Unforgettable Understanding of the Python Programming Language.
- Gain the Python Skills Necessary to Learn In-Demand Topics, such as Data Science, Web Development, AI and more.
- Build 11 Fun and Memorable Python Projects.
- Use Logic and Data Structures to Create Python Programs That Can Think.
- Use Object-Oriented Programming (An Industry-Standard Coding Technique) to Write High Quality Python Code.
- Use Raw Text Data in Python to Generate Automated Messages and Customise User Experiences.
- Use Loops in Python to Improve Code Efficiency and Maximize Your Productivity.
- Create Your Own Custom Python Functions to Simplify Your Code.
Machine Learning A-Z™: Hands-On Python & R In Data Science
- Master Machine Learning on Python & R
- Have a great intuition of many Machine Learning models
- Make accurate predictions
- Make powerful analysis
- Make robust Machine Learning models
- Create strong added value to your business
- Use Machine Learning for personal purpose
- Handle specific topics like Reinforcement Learning, NLP and Deep Learning
- Handle advanced techniques like Dimensionality Reduction
- Know which Machine Learning model to choose for each type of problem
- Build an army of powerful Machine Learning models and know how to combine them to solve any problem
Best Python Books
Automate the Boring Stuff with Python, 2nd Edition: Practical
The second edition of this best-selling Python book (100,000+ copies sold in print alone) uses Python 3 to teach even the technically uninclined how to write programs that do in minutes what would take hours to do by hand. There is no prior programming experience required and the book is loved by liberal arts majors and geeks alike.
Python Cookbook: Recipes For Mastering Python, Third Edition
If you need help writing programs in Python 3or want to update older Python 2 code, this book is just the ticket. Packed with practical recipes written and tested with Python 3.3, this unique cookbook is for experienced Python programmers who want to focus on modern tools and idioms.
Inside, you’ll find complete recipes for more than a dozen topics, covering the core Python language as well as tasks common to a wide variety of application domains. Each recipe contains code samples you can use in your projects right away, along with a discussion about how and why the solution works.
Zed Shaw has perfected the world’s best system for learning Python 3. Follow it and you will succeed―just like the millions of beginners Zed has taught to date! You bring the discipline, commitment, and persistence; the author supplies everything else.
In Learn Python 3 the Hard Way, you’ll learn Python by working through 52 brilliantly crafted exercises. Read them. Type their code precisely. (No copying and pasting!) Fix your mistakes. Watch the programs run. As you do, you’ll learn how a computer works; what good programs look like; and how to read, write, and think about code. Zed then teaches you even more in 5+ hours of video where he shows you how to break, fix, and debug your code―live, as he’s doing the exercises.
What exactly is machine learning and why is it so valuable in the online business world? Simply put, it is a method of data analysis that uses algorithms that learn from data and produce specific results without being specifically programmed to do so. These algorithms can analyze data, calculate how frequently certain parts of it are used and generate responses based on this calculations in order to automatically interact with users.
Throughout the recent years, artificial intelligence and machine learning have made some enormous, significant strides in terms of universal, global applicability. The ability to now be able to apply such algorithms via traditional and new dynamic programming languages allows us to interpret and understand these approaches much more eloquently.
Python for Software Design is a concise introduction to software design using the Python programming language. Intended for people with no programming experience, this book starts with the most basic concepts and gradually adds new material. Some of the ideas students find most challenging, like recursion and object-oriented programming, are divided into a sequence of smaller steps and introduced over the course of several chapters. The focus is on the programming process, with special emphasis on debugging.
he book includes a wide range of exercises, from short examples to substantial projects, so that students have ample opportunity to practice each new concept. Exercise solutions and code examples are available from thinkpython.com, along with Swampy, a suite of Python programs that is used in some of the exercises.
Best Python IDEs
Best Websites to Learn Python
Do you code regularly ? Read this trick to boost your coding skills !
Best Python Communities
Best Python Youtube Channels
Best Python Repositories
Best Python Blogging Platforms
Best Online Python Compilers
Best Python Newsletters
- Awesome Python Newsletter
- Python Weekly
- The Real Python Newsletter
- Become a Better Developer
- Bite Python
End Notes
The python language is one of the most accessible programming languages available because it has simplified syntax and not complicated, which gives more emphasis on natural language. Due to its ease of learning and usage, python codes can be easily written and executed much faster than other programming languages. Hope you have a good python learning experience.
All the best !
Disclaimer : All these projects are sourced from various blogs over the internet, stack overflow, portals like geekforgeeks, hashnode and more. If you think it is your work and need to be given due credit, please write to aerospace.prince@gmail.com and I’ll update. Thanks !
There is definately a lot to know about this subject. I love all of the points you have made. Joleen Ezechiel Broderick